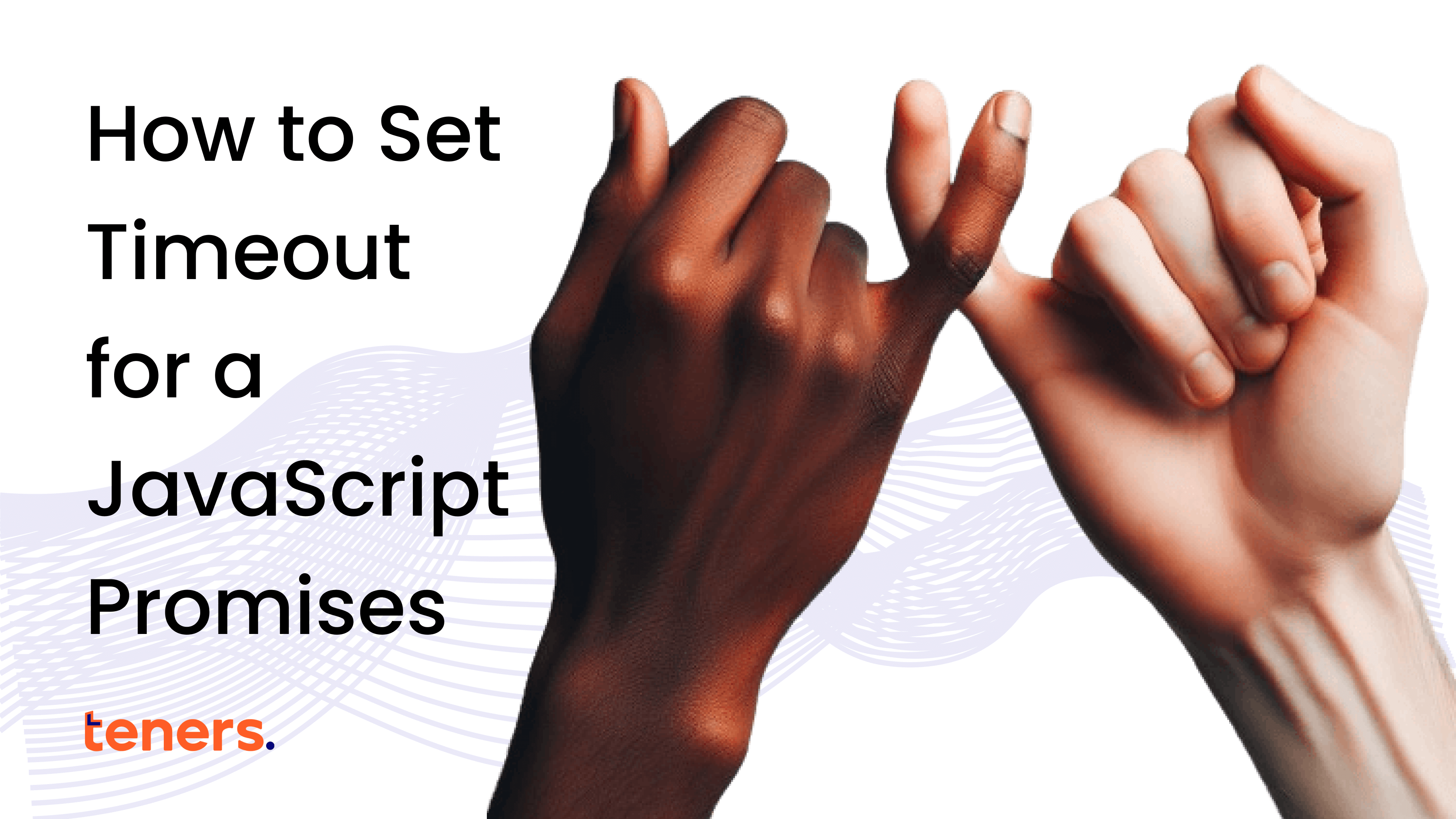
JavaScript guarantees are a robust instrument for dealing with asynchronous code execution. They permit us to put in writing cleaner and extra maintainable code by making it simpler to deal with async operations. Nevertheless, there are occasions after we wish to set a timeout for a promise to verify it does not take too lengthy to resolve or reject. On this article, we’ll discover completely different approaches to setting a timeout for a JavaScript promise and talk about the professionals and cons of every.
Strategy 1: Utilizing Promise.race
One method to set a timeout for a promise is through the use of the Promise.race
methodology. This methodology takes an array of guarantees and returns a brand new promise that’s settled as quickly as any of the enter guarantees are settled. We are able to leverage this habits to create a timeout mechanism. This is an instance:
perform timeoutPromise(promise, timeout) {
const timeoutPromise = new Promise(
(resolve, reject) => {
setTimeout(() => {
reject(new Error('Promise timed out'))
}, timeout)
}
);
return Promise.race([promise, timeoutPromise]);
}
// Utilization
const promise = new Promise((resolve, reject) => {
// some async operation
});
const timeout = 5000; // 5 seconds
const consequence = await timeoutPromise(promise, timeout);
On this instance, we create a brand new promise known as timeoutPromise
that rejects after the required timeout. We then use Promise.race
to return a brand new promise that settles both with the results of the unique promise or with a timeout error, whichever happens first.
Strategy 2: Utilizing async/await and setTimeout
One other method is to leverage the ability of async/await
syntax together with setTimeout
to create a timeout mechanism for a promise.
perform timeoutPromise(promise, timeout) {
return new Promise(
async (resolve, reject) => {
const timeoutId = setTimeout(() => {
clearTimeout(timeoutId);
reject(new Error('Promise timed out'));
}, timeout);
strive {
const consequence = await promise;
clearTimeout(timeoutId);
resolve(consequence);
}
catch (error) {
clearTimeout(timeoutId);
reject(error);
}
}
);
}
// Utilization
const promise = new Promise((resolve, reject) => {
// some async operation
});
const timeout = 5000; // 5 seconds
const consequence = await timeoutPromise(promise, timeout);
Right here, we create a brand new promise that wraps the unique promise. We use async/await
to deal with the asynchronous nature of the unique promise. We begin a timer utilizing setTimeout
, and if the timer expires earlier than the unique promise resolves or rejects, we reject the brand new promise with a timeout error. In any other case, we clear the timer and resolve or reject the brand new promise primarily based on the end result of the unique promise.
Each of those approaches obtain the purpose of setting a timeout for a JavaScript promise. Nevertheless, they differ in how they deal with the timeout mechanism. In method 1 we use a Promise.race
to race the unique promise with a timeout promise, whereas method 2 makes use of setTimeout together with async/await to create a extra built-in and readable resolution.
It is vital to notice that setting a timeout for a promise is just not a assure that the underlying async operation might be canceled. It solely ensures that the promise itself will settle throughout the specified timeout.
If obligatory, it is best to deal with the cleanup of any sources related to the async operation individually.
Conclusion
Setting a timeout for a JavaScript promise might be achieved utilizing completely different approaches. The selection relies on the necessities and elegance preferences of the mission. Whatever the method you select, including a timeout to guarantees can vastly improve the robustness and reliability of your asynchronous code.